Advance Your Business in the 4th Revolution
We're in the midst of the Fourth Tech Revolution, powered by Generative AI, and there's no better moment to elevate your business. Trust the enterprise app development team that has served businesses through the last 3 tech revolutions.
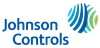
Join our happy and
satisfied customers.
Better AI Software Faster
Over 20 years we earned a reputation of expeditious dev cycles with very high quality software due to our unique development process.
We've done it again in the age of Generative AI. Over the last several years we've developed a propriety AI development process, that gives the edge in these areas:
Speed!
Oh, have we got speed! We use a proprietary blend of AI Agents and Custom Prompt Engineering and other secret sauce to achieve dev speeds exceeding 5x of previously already high standards.
Quality
We use best in class observation and evaluation tools with agent as a judge and human in the loop test cycles to ensure dialed in deliveries.
Security
Our siloed agent architecture and strict guardrail testing achieve standards our HIPAA clients require. You can count on our prudent approach to safety and security.
Better: Part Deux
We've seen the web revolution, the mobile revolution, the cloud revolution... And now the AI revolution is here. And this one is important for your business by orders of magnitude!
Don't get left behind.
Modern Enterprise Web Applications
Modern web applications are built with the latest technologies and best practices. We build scalable, secure, and responsive web applications that are easy to maintain and provide a great user experience. Whether you are a startup or a Fortune 500 company, we've seen it and done it all. But now we get to do it faster and better than ever before with our proprietary AI development process.
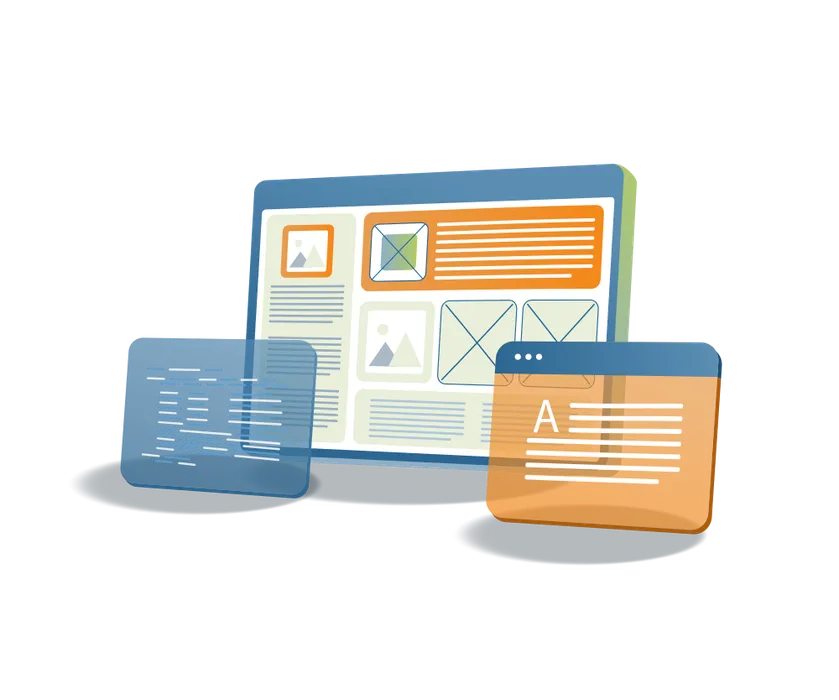
Efficient Development Methodology
Efficient development methodologies are used to deliver high-quality software on time and on budget. We use agile development practices, continuous integration, and automated testing to ensure quality and speed. Now turbocharged with AI.
AI Enhanced User Experiences
AI enhanced user experiences provide personalized content, recommendations, and interactions. We use machine learning algorithms to analyze user data and provide a customized experience that improves engagement and satisfaction.
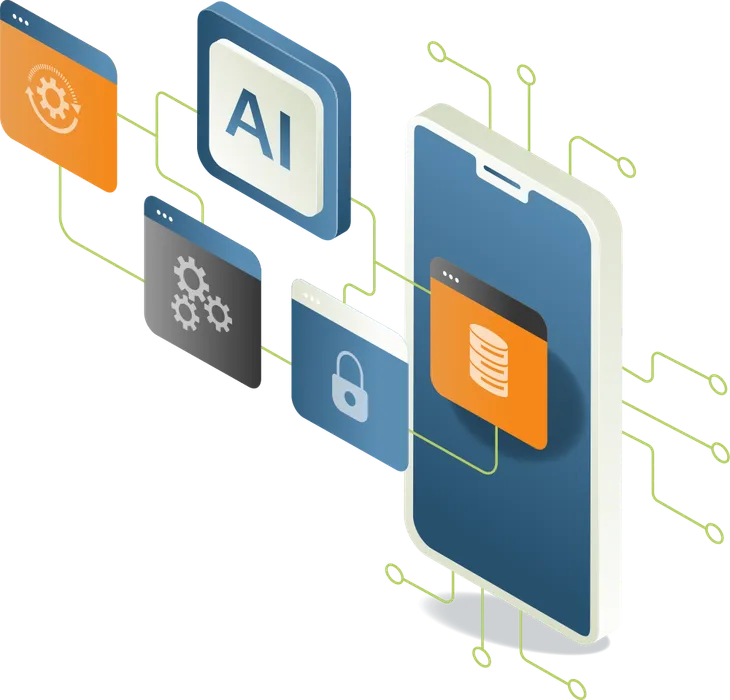
Powerful AI Integrations Improve User Experience
Supercharge your new or current application with integrated AI!
Customer Service Chat
Customer facing chat bots can help answer questions, provide information, and assist with common tasks. Trained with your company data, chat bots improve customer service, reduce response times, and enhance customer satisfaction.
Task Automation
Automate routine tasks with an AI Assistant. For example, customer support emails scanned by an AI Assistant are categorized as a suggestion, question or complaint. A draft email is composed by the AI assistant and queued for human approval - substantially reduce reply time and minimize manual work.
Intelligent Form Filling Autocompletion
Use artificial intelligence to predict and complete form fields based on user input and historical patterns. Speed up form completion, reduce errors, and improve user experience.
Data Extraction
Identify semantically important details such as names, dates, addresses, and figures in uploaded documents, forms, invoices, and more. The extractions can be used to automate data entry, improve data accuracy, and reduce manual work.
Voice-to-Text Data Entry
AI assisted speech recognition can transcribe audio recordings, dictate text, and automate data entry processes. Whether on the office laptop or on the go with a mobile device, voice-to-text data entry can save time and improve productivity.
Predictive Analytics
Business intelligence tools can be used to analyze data, identify trends, and make predictions about future outcomes. Predictive analytics can be used to forecast sales, optimize marketing campaigns, and improve decision-making processes.
Our customers love us
Trusted by Industry Leaders
We've helped organizations of all sizes achieve their digital transformation goals.
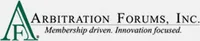
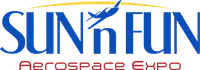
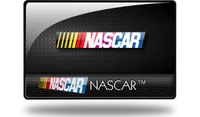
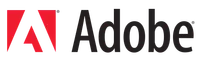
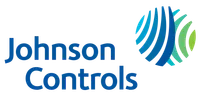
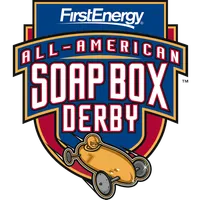
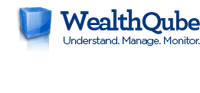
What are you waiting for? Let's talk today!
See how efficient your product can be.